关于Git与GitHub的记录
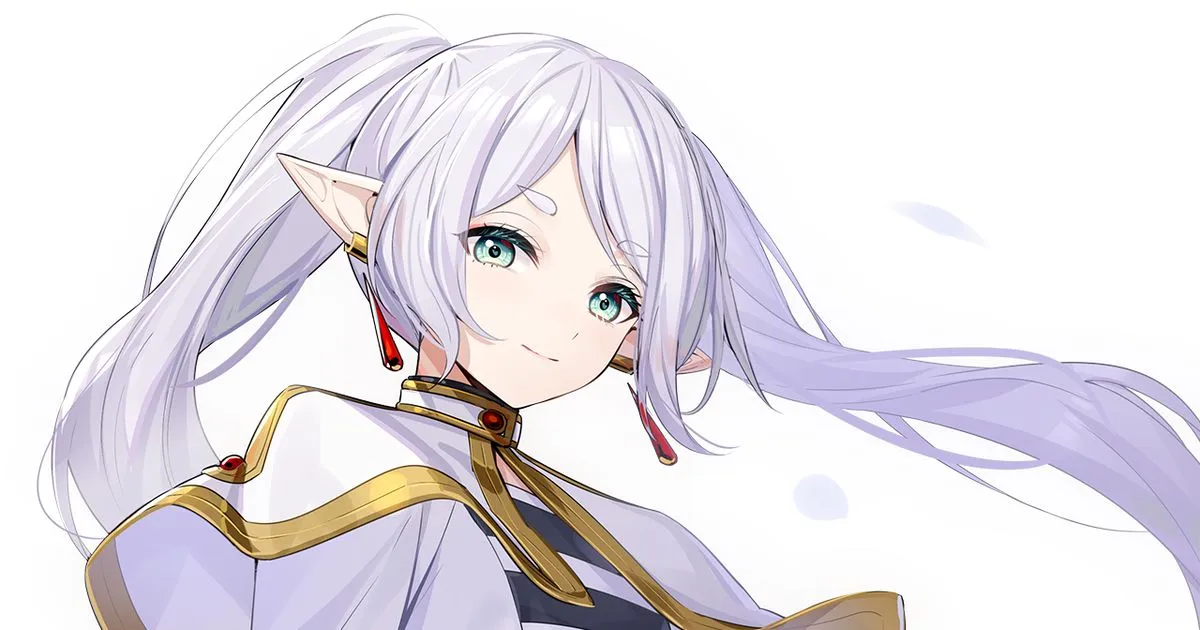
关于git的资料
关于git的资料
- https://www.atlassian.com/git
- https://nulab.com/zh-cn/learn/software-development/git-tutorial/
- https://learngitbranching.js.org/?locale=zh_CN 可视化git
- https://git-scm.com/book/en/v2
常用命令
- 配置 Git 环境:
git config -l
: 查看当前 Git 配置信息。git config --global user.name "Your Name"
: 设置全局用户名。git config --global user.email "your.email@example.com"
: 设置全局用户邮箱。
- 基本操作:
git init
: 在当前目录初始化一个 Git 仓库。git add <file>
: 将文件添加到暂存区。git rm --cached <file>
: 从暂存区中移除文件。git commit -m "Commit message"
: 提交暂存区中的文件到本地仓库。
- 查看状态和日志:
git status
: 查看工作区和暂存区的状态。git log
: 查看提交历史。git log --graph --oneline
: 查看简洁的提交历史图。
- 远程仓库操作:
git remote add origin <url>
: 关联远程仓库。git push -u origin master
: 推送本地仓库内容到远程仓库。git clone <url> <directory>
: 克隆远程仓库到本地。git pull origin master
: 拉取远程仓库内容到本地。
- 分支操作:
git branch
: 显示分支列表。git branch <branch-name>
: 创建新分支。git checkout <branch-name>
: 切换到指定分支。git merge <branch-name>
: 将指定分支合并到当前分支。git branch -d <branch-name>
: 删除指定分支。
- 高级操作:
git rebase master
: 将当前分支的提交整合到主分支。git rebase --continue
: 在解决冲突后继续 rebase 操作。git rebase --abort
: 取消正在进行的 rebase 操作。
- 其他命令:
git fetch
: 从远程仓库获取最新内容。git tag
: 打标签并管理标签。git grep <pattern>
: 在文件中搜索指定内容。git grep -n <pattern>
: 显示搜索结果行号。
rebase 与 merge
git rebase
适合用于合并一些临时性的分支或者个人开发分支,它可以将这些分支的提交整理成一个线性的提交历史,保持提交历史的干净和整洁,并且不会产生额外的合并提交。这样的操作适合于个人开发或者小团队开发的场景。
git merge
则更适合用于合并一些功能性分支或者长期稳定的分支,它会在目标分支上创建一个新的合并提交,将源分支的修改合并进来。这样的操作会保留分支之间的关系,形成一个分叉的提交历史结构,适合于多人协作或者长期开发的场景。
具体使用的话总结: 子分支 rebase master , master merge 子分支
多账号
一台电脑多账号的情况下区分各自仓库
查看全局配置
|
|
清除全局配置
|
|
生成新密钥
|
|
~/.ssh/
现在得到了:
|
|
- 私钥
- 公钥绑定到GitHub
将私钥添加到ssh代理
|
|
关于ssh代理看这里
将公钥添加到GitHub
打开github.com, 设置 > SSH 和 GPG 密钥 > 新 SSH 密钥
将之前的后缀为.pub
的公钥复制 , 添加进去
配置文件
现在到~/.ssh/config
如果没有就新建一个
|
|
在config
中写入
|
|
推送至各自仓库
现在本地没有全局user.email和user.name
所以各自账号的仓库都需要手动的配置一次
|
|
现在添加远程仓库需要改动一些内容
这是原来的
|
|
改成
|
|
- Host 就是
config
文件里你配置的 Host
eg:
|
|
测试与GitHub是否连通
|
|
-
如果返回
kex_exchange_identification: Connection closed by remote host
可以更改config配置
-
配置1
- 不指定端口
- HostNmae使用
github.com
|
|
- 配置2
- 指定443端口
- HostNmae使用
ssh.github.com
|
|
这时候再次进行测试
|
|
这样就完成了!
疑难杂症
22端口超时
Q: # [ssh: connect to host github.com port 22: Connection timed out](https://stackoverflow.com/questions/15589682/ssh-connect-to-host-github-com-port-22-connection-timed-out)
A:
|
|